mirror of
https://github.com/excaliburpartners/OmniLinkBridge
synced 2024-12-22 18:52:24 +00:00
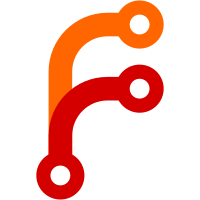
- Added additional zone types to contact and motion web service API - Split command line options for config and log files
60 lines
2 KiB
C#
60 lines
2 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Net;
|
|
|
|
namespace HAILogger
|
|
{
|
|
static class WebNotification
|
|
{
|
|
private static List<string> subscriptions = new List<string>();
|
|
private static object subscriptions_lock = new object();
|
|
|
|
public static void AddSubscription(string callback)
|
|
{
|
|
lock (subscriptions_lock)
|
|
{
|
|
if (!subscriptions.Contains(callback))
|
|
{
|
|
Event.WriteVerbose("WebNotification", "Adding subscription to " + callback);
|
|
subscriptions.Add(callback);
|
|
}
|
|
}
|
|
}
|
|
|
|
public static void Send(string type, string body)
|
|
{
|
|
string[] send;
|
|
lock (subscriptions_lock)
|
|
send = subscriptions.ToArray();
|
|
|
|
foreach (string subscription in send)
|
|
{
|
|
WebClient client = new WebClient();
|
|
client.Headers.Add(HttpRequestHeader.ContentType, "application/json");
|
|
client.Headers.Add("type", type);
|
|
client.UploadStringCompleted += client_UploadStringCompleted;
|
|
|
|
try
|
|
{
|
|
client.UploadStringAsync(new Uri(subscription), "POST", body, subscription);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
Event.WriteError("WebNotification", "An error occurred sending notification to " + subscription + "\r\n" + ex.ToString());
|
|
subscriptions.Remove(subscription);
|
|
}
|
|
}
|
|
}
|
|
|
|
static void client_UploadStringCompleted(object sender, UploadStringCompletedEventArgs e)
|
|
{
|
|
if (e.Error != null)
|
|
{
|
|
Event.WriteError("WebNotification", "An error occurred sending notification to " + e.UserState.ToString() + "\r\n" + e.Error.Message);
|
|
|
|
lock (subscriptions_lock)
|
|
subscriptions.Remove(e.UserState.ToString());
|
|
}
|
|
}
|
|
}
|
|
} |